Database Management with Eloquent ORM in Laravel
Learn how to use the Laravel ORM to connect to databases
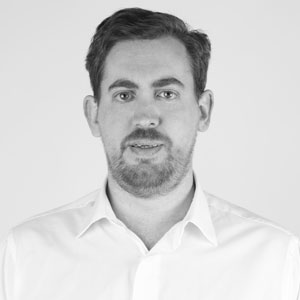
Database management is an essential aspect of any web application. Laravel, a popular PHP framework, makes this task easy with Eloquent ORM (Object-Relational Mapping). Eloquent is an efficient and elegant way to interact with databases using objects and relationships instead of directly writing SQL queries.
What is an ORM?
ORM stands for "Object-Relational Mapping" or "Object-Relational Mapping". It is a programming technique used to convert data between the type system used in an object-oriented programming language and the type system used in a relational database.
In simpler terms, an ORM is a way to interact with your database, just as if it were an object-oriented database.
Benefits of using an ORM
Here are some benefits of using an ORM when developing software:
- Database Abstraction : ORMs allow developers to write queries using the programming language they are working with, rather than SQL or some other database query language. This means you can focus on writing code in a language you already know, which can increase your productivity.
- Database system independence : With an ORM, the code you write can be independent of the underlying database system. This means that you can switch to a different database system with minimal code changes.
- Faster Development – ORMs often come with many built-in features such as query handling, transactions, migrations, seeds, and more. These features can help you develop faster by saving you the need to write this code manually.
- Security : ORMs often have built-in protections against common database attacks, such as SQL injection. This can make your app more secure.
- Integration with object-oriented code : Since ORMs allow you to interact with your data as objects, you can use all the power of object-oriented programming, such as inheritance, encapsulation, polymorphism, etc.
- Maintainability : Code is generally easier to read and understand, which can make maintaining and updating your app much easier.
It's important to note that while ORMs have many benefits, they can also have drawbacks, such as additional overhead and loss of control over database queries in some cases. However, for many applications, the benefits of using an ORM far outweigh these disadvantages.
What is Eloquent ORM?
Eloquent ORM is a technique that allows you to interact with databases in Laravel as if they were PHP objects. ORM stands for "Object-Relational Mapping" and is a programming paradigm that converts data between incompatible systems using "objects". This technique makes data manipulation easier and reduces the need to write SQL code.
Eloquent Configuration
To start using Eloquent, we will need to define models. In Laravel, every table in the database has a corresponding "Model" that is used to interact with that table.
An Eloquent model is simply a PHP class found in the app/ directory by default. To create a new model, you can use the artisan make:model command. For example, for a users table, you can create a User model with the following command:
php artisan make:model User
This will create a new model class in app/Models/User.php.
CRUD (Create, Read, Update, Delete) with Eloquent
With Eloquent, CRUD operations (Create, Read, Update, Delete) are easy.
create
To create a new record in the database, simply create a new instance of the model, set its attributes, and then call the save method.
$user = new User;
$user->name = 'John Doe';
$user->email = 'john@example.com';
$user->password = Hash::make('password');
$user->save();
Read
To read data, you can use various methods available in Eloquent. For example, to get all users, you can do the following:
$users = User::all();
Updating a record is as easy as changing a few attributes and then calling the save method.
$user = User::find(1);
$user->email = 'new-email@example.com';
$user->save();
delete
Finally, to delete a record, call the delete method.
$user = User::find(1);
$user->delete();
Relations with Eloquent
Eloquent also makes it easy to define and manage relationships between tables, such as "one-to-one", "one-to-many", "many-to-many", "has-one-through", "has-many-through", and "many-to-many". polymorphic".
For example, if a User has many Posts, you can define this relationship in the User model as follows:
public function posts()
{
return $this->hasMany('App\Models\Post');
}
Then, you can access all the posts of a user as follows:
$user = User::find(1);
$posts = $user->posts;
In summary, Eloquent ORM in Laravel offers an intuitive and flexible interface to handle interaction with databases. Its object-oriented approach and wide range of functionality make database management much more manageable and less error-prone.
Advanced Queries
In addition to basic CRUD operations, Eloquent offers a large number of methods for performing more complex queries. For example, if you wanted to get all users with more than 100 posts, you could do it like this:
$users = User::has('posts', '>', 100)->get();
Or if you wanted to get all of a user's posts that were created in the last month, you could do it like this:
$recentPosts = $user->posts()->where('created_at', '>', Carbon::now()->subMonth())->get();
complex functions
Join
To perform a join operation, you can use the join method in an Eloquent query constructor. For example, if you wanted to get all the users along with their post details, you could do it like this:
$users = DB::table('users')
->join('posts', 'users.id', '=', 'posts.user_id')
->select('users.*', 'posts.description')
->get();
Here, we are joining the 'users' and 'posts' tables where the 'id' from the 'users' table matches the 'user_id' from the 'posts' table.
Union
To combine the results of multiple queries into a single result set, you can use the union method. For example, if you wanted to get all the posts that were published today or that have at least 10 likes, you could do it like this:
$first = DB::table('posts')
->where('created_at', '=', Carbon::today());
$posts = DB::table('posts')
->where('likes', '>=', 10)
->union($first)
->get();
Group By
The groupBy method allows you to group the query results by one or more columns. For example, if you wanted to get the number of posts each user has created, you could do it like this:
$users = DB::table('posts')
->select('user_id', DB::raw('count(*) as total_posts'))
->groupBy('user_id')
->get();
Having
The having method allows you to filter the query results by an SQL "having" condition. For example, if you wanted to get only the users who have created more than 10 posts, you could do it like this:
$users = DB::table('posts')
->select('user_id', DB::raw('count(*) as total_posts'))
->groupBy('user_id')
->having('total_posts', '>', 10)
->get();
Remember that these are only the basic operations that you can perform with Eloquent. Laravel offers a lot of additional functionality that can help you build more complex and powerful queries.
Mutators and Accessors
Eloquent also provides functionality for mutators and accessors, which allow you to define how a model's attributes should be manipulated when they are set or obtained. For example, if you wanted to ensure that all user emails are in lowercase, you could define a mutator on the User model as follows:
public function setEmailAttribute($value)
{
$this->attributes['email'] = strtolower($value);
}
Conclusion
Eloquent ORM is a powerful and flexible tool for managing databases in Laravel. Its object-oriented approach and wide range of functionality make database management much more manageable and enjoyable. By learning to use Eloquent, you can greatly improve your efficiency and productivity as a Laravel developer.